2022. 7. 18. 14:12ㆍDEV/Flutter
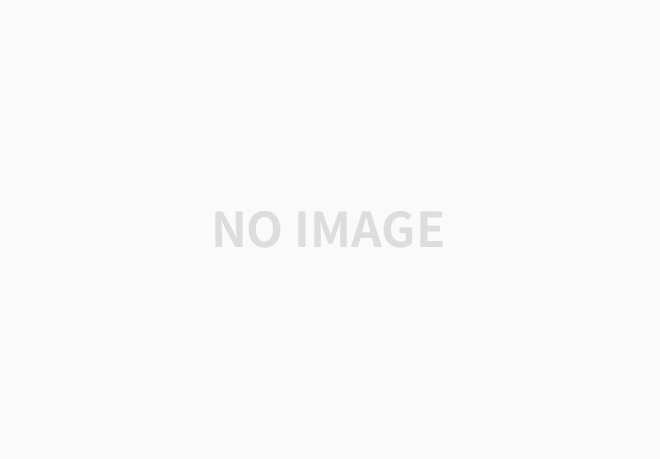
시작 전 참고
Firebase Authentication 설정
Firebase Console에 접속 후 프로젝트 페이지로 이동한다.
Authentication 시작
Console > Project > Authentication > 시작하기
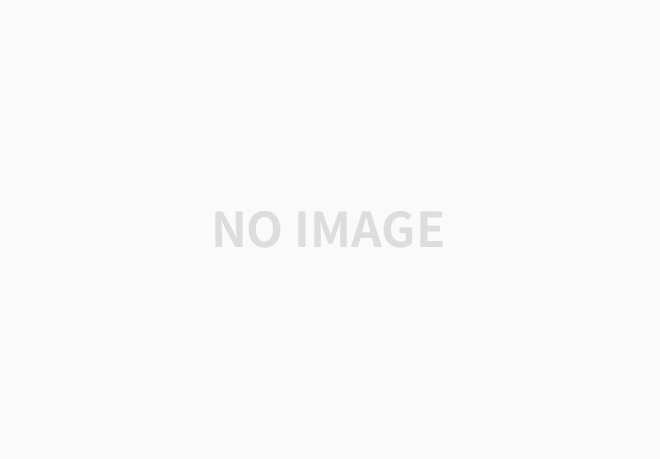
이메일 사용 설정
Authentication > Sign-in method > 이메일/전화번호
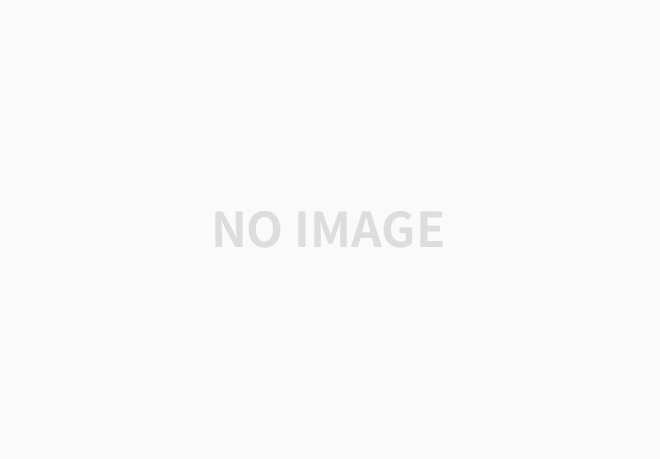
이메일/비밀번호 사용 설정을 활성화시킨 뒤 저장을 누른다.
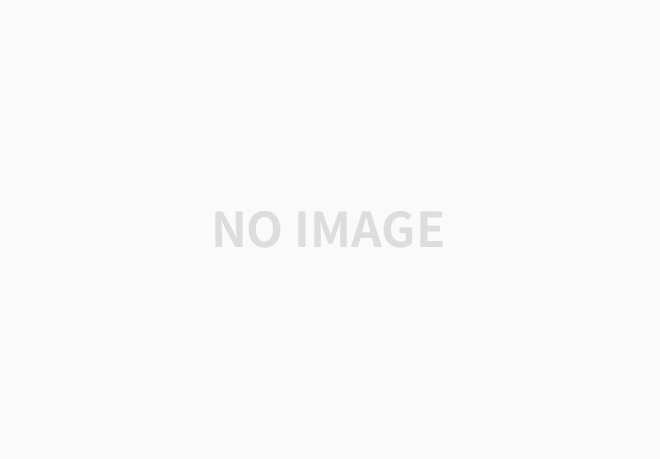
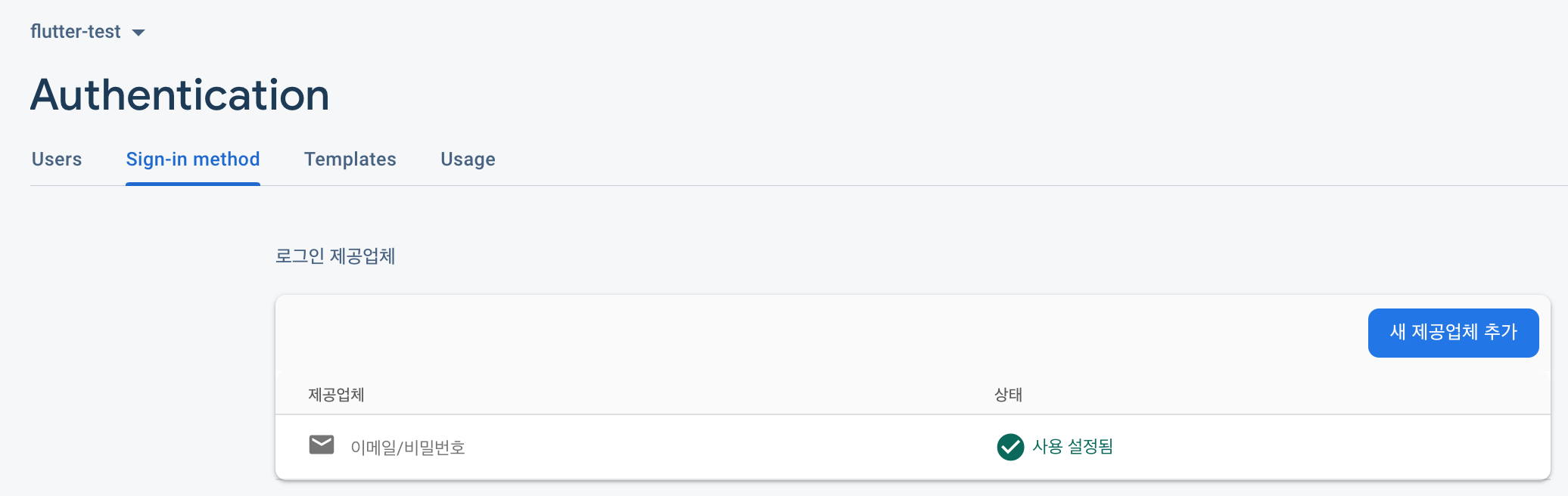
Firebase로 회원 가입하기
일단 이메일로 가입하는 기능을 구현해보자.
Flutter에서 기능 구현하기
Firebase Authentication 인스턴스 생성
Firebase Authenticaton을 사용하기 위해 Auth의 인스턴스를 생성한다.
class _SignScreenState extends State<SignScreen> {
final _auth = FirebaseAuth.instance;
...
}
회원 가입 실행
email & password로 회원가입하기를 해보자.
Button을 클릭했을 때 Form 데이터로 가입을 실행하도록 코드를 작성한다. 가입한 결과를 User Type의 변수에 담아서 가입 성공 여부판단의 기준으로 사용한다.
이때 Cloud Firestore 데이터에 가입한 사용자의 정보를 저장시켜보자.
💡 FIrebase 사용 설정이 되어 있다면 user 컬렉션을 미리 생성하지 않아도 저장 시 생성까지 처리가 된다.
ElevatedButton(
...
onPressed: () async {
// * Validation
if (_formKey.currentState!.validate()) {
// * 입력된 값들을 모두 state에 저장하도록 각 Field의 onSaved를 실행
_formKey.currentState!.save();
}
try {
// * Email & Password로 회원 가입
final credential = await _auth.createUserWithEmailAndPassword(
email: email, password: password);
// * 가입완료한 user의 정보를 제대로 받은 경우
if (credential.user != null) {
// * user의 정보를 데이터 베이스에 등록
await FirebaseFirestore.instance
.collection('user')
.doc(credential.user!.uid)
.set({
// .set(<String, dynamic>{
'userName': name,
'email': email,
});
// * 기타 Widget 관련 처리
setState(() {
// * Reset password field
_pwdController.clear();
// * Sign In으로 변경
isSignIn = true;
});
}
} on FirebaseAuthException catch (e) {
ScaffoldMessenger.of(context)
.showSnackBar(SnackBar(content: Text(e.message!)));
}
}
가입한 정보 받아오기
가입 정보를 사용할 클래스에서 다음과 같이 정보를 받아서 사용할 수 있다.
final _authentication = FirebaseAuth.instance;
User? loggedUser; // * 등록된 유저가 없을 수 있으므로 Nullable
void getCurrentUser() {
try {
// * 등록된 유저를 받아오기
final user = _authentication.currentUser;
if (user != null) {
loggedUser = user;
}
print(user!.email);
} catch (e) {
print(e);
}
}
Firebase 가입 결과 확인
Authentication > Users
Firebase 콘솔에서 가입된 정보를 확인할 수 있다.
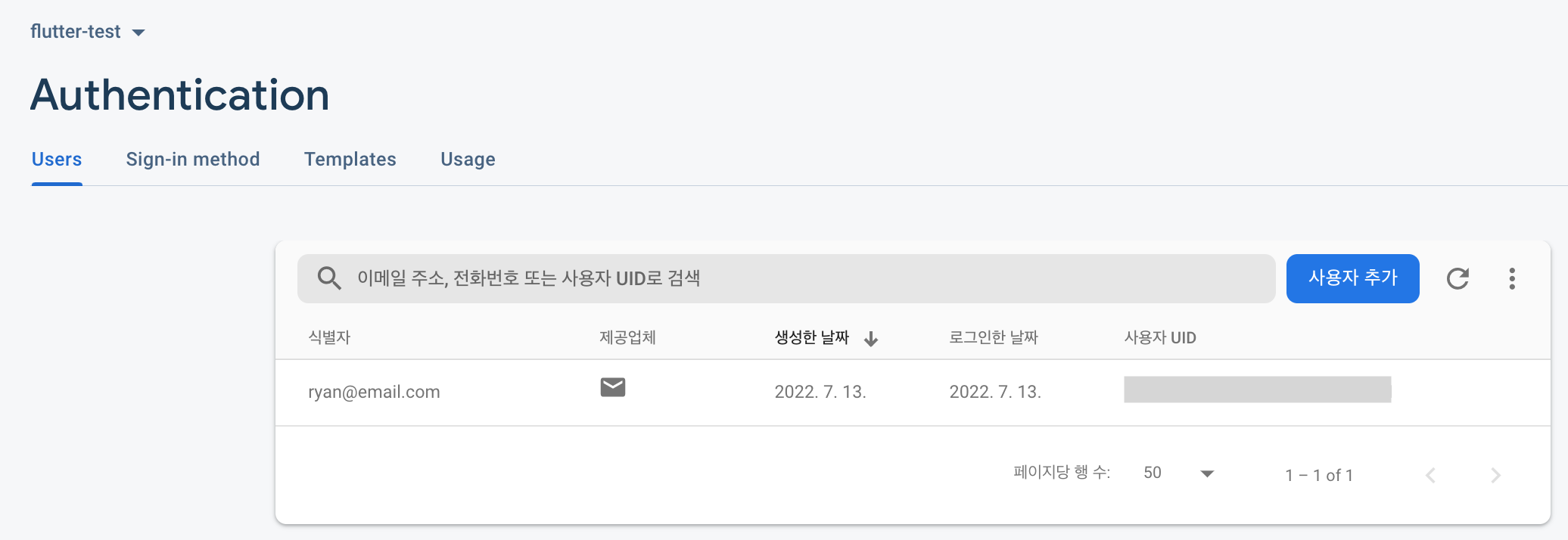
Firebase로 로그인하기
회원가입할 때와 마찬가지로 간단한 함수 하나면 된다.
try {
final credential = await _auth.signInWithEmailAndPassword(
email: email, password: password);
if (credential.user != null) {
// * Sign in Success -> Go to Chat Page
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => const ChatScreen(),
),
);
}
} on FirebaseException catch (e) {
// * Firebase Error Handling
ScaffoldMessenger.of(context)
.showSnackBar(SnackBar(content: Text(e.message!)));
} catch (e) {
// * Other Error Handling
print(e);
}
=>
UserCredential(additionalUserInfo: AdditionalUserInfo(isNewUser: false, profile: {}, providerId: null, username: null), credential: null, user: User(displayName: null, email: ryan@email.com, emailVerified: false, isAnonymous: false, metadata: UserMetadata(creationTime: 2022-07-18 00:32:33.388, lastSignInTime: 2022-07-18 13:00:01.580), phoneNumber: null, photoURL: null, providerData, [UserInfo(displayName: null, email: ryan@email.com, phoneNumber: null, photoURL: null, providerId: password, uid: ryan@email.com)], refreshToken: , tenantId: null, uid: 3RB17NhxWURqxJwQsXmvbPdSsvo2))
Firebase 로그 아웃
일단 여기에서는 authStateChanges를 사용해서 Authenctication State를 구독하도록 하자.
Sing out Button
onPressed: () {
_auth.signOut();
}
main.dart
Authentication State에 따라 보여줄 화면 설정을 한다.
...
home: StreamBuilder(
stream: FirebaseAuth.instance.authStateChanges(),
builder: (BuildContext context, AsyncSnapshot<User?> snapshot) {
if (snapshot.hasData) {
// * 인증된 데이터가 있다면 채팅 화면으로 이동
return const ChatScreen();
}
// * 없으면 로그인 화면으로 이동
return const SignScreen();
},
)
REF
🔗 Authenticate with Firebase using Password-Based Accounts on Flutter
'DEV > Flutter' 카테고리의 다른 글
[Firebase & iOS Xcode Build Error] Cloud Firestore Package 설치 후 Xcode Build가 너~~무 느려서 진행이 안되는 문제 해결 방법 (0) | 2022.08.17 |
---|---|
Android Emulator에서 한글 키보드 사용하기 (0) | 2022.07.23 |
Flutter에서 Firestore Database 사용하기 (0) | 2022.07.20 |
Flutter & Firebase - Authentication State 구독 메소드 (0) | 2022.07.18 |
Flutter & Firebase - iOS 오류 (0) | 2022.07.15 |
Flutter & Firebase - Flutter multidex handling is disabled 오류 해결 방법 (0) | 2022.07.15 |
Flutter & Firebase Android SDK Version 오류 해결 (0) | 2022.07.15 |
Flutter 프로젝트에 Firebase 연동하기 (0) | 2022.07.13 |